Introduction
Java is a versatile object-oriented programming language, known for its simplicity and flexibility. One important concept within Java's inheritance model is Multiple Hierarchical Inheritance, which may seem complex at first but can be understood with a little effort. In this article, we will break down the concept of multiple hierarchical inheritance and how it works in Java, simplifying it for beginners.
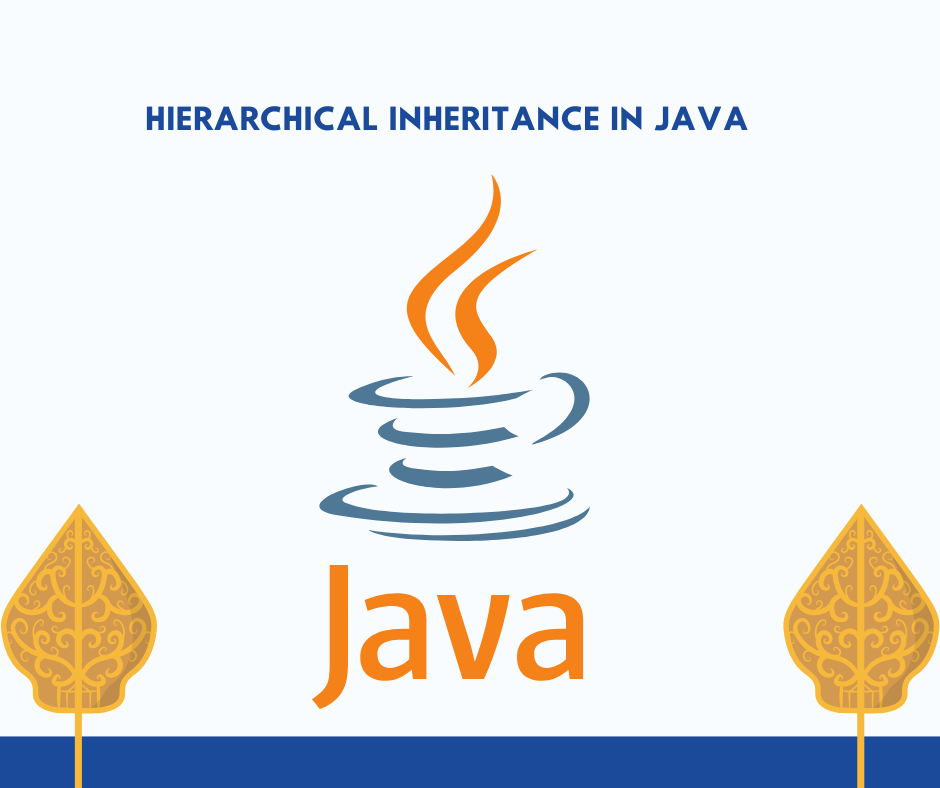
What is Inheritance in Java?
Before diving into multiple hierarchical inheritance, let's first understand the basic idea of inheritance in Java. Inheritance is one of the core principles of object-oriented programming (OOP). It allows a class (called the subclass or child class) to inherit properties and behaviors (fields and methods) from another class (called the superclass or parent class). This establishes a natural structure and encourages code reuse.
Types of Inheritance in Java
In Java, there are mainly two types of inheritance:
Single Inheritance: This is where a class inherits from only one parent class.
Multiple Inheritance: This occurs when a class inherits from more than one class. However, Java does not support multiple inheritance with classes directly due to ambiguity issues.
But, Java allows Multiple Hierarchical Inheritance through its class and interface mechanism.
What is Multiple Hierarchical Inheritance?
Multiple Hierarchical Inheritance refers to the scenario where a single class (child class) inherits from more than one parent class, creating a hierarchy.
Hierarchical inheritance happens when a single parent class has multiple child classes.
Multiple inheritance occurs when a class can inherit from more than one class.
In Java, you cannot directly inherit from multiple classes, but you can simulate this behavior using interfaces. Let's understand this in more detail.
How Java Implements Multiple Hierarchical Inheritance?
Java does not support multiple inheritance with classes because it can lead to ambiguity. For instance, if two parent classes have a method with the same signature, it becomes unclear which method the child class should inherit.
However, Java allows Multiple Hierarchical Inheritance by using interfaces. Like a class, an interface is a reference type that can only include nested types, default methods, static methods, constants, and method signatures. Instance fields are not allowed in interfaces, and all methods—aside from default and static methods—are abstract by default. Here's a simple breakdown of how Java implements multiple hierarchical inheritance using interfaces:
A class can implement multiple interfaces.
An interface can extend other interfaces, forming a hierarchical structure.
Example of Multiple Hierarchical Inheritance in Java Using Interfaces
Let's illustrate multiple hierarchical inheritance with a practical example:
java
Copy code
// Defining the first interface
interface Animal {
void sound(); // Method abstraction
}
// Defining the second interface
interface Mammal {
void walk(); // Abstract method
}
// Child class that implements both interfaces
class Dog implements Animal, Mammal {
// Implementing the sound method from Animal interface
public void sound() {
System.out.println("Dog barks");
}
// Implementing the walk method from Mammal interface
public void walk() {
System.out.println("Dog walks on four legs");
}
}
public class Main {
public static void main(String[] args) {
// Creating an instance of Dog
Dog dog = new Dog();
dog.sound(); // Outputs: Dog barks
dog.walk(); // Outputs: Dog walks on four legs
}
}
In this example, the Dog class implements two interfaces: Animal and Mammal. The Animal interface has the sound() method, while the Mammal interface has the walk() method. The Dog class provides implementations for both methods.
Advantages of Multiple Hierarchical Inheritance in Java
Code Reusability: By inheriting behavior from multiple interfaces, Java allows classes to reuse code without the risk of redundancy.
Modularity: Interfaces provide a way to modularize the code. You can define specific behaviors in separate interfaces and implement them in the class as needed.
Flexibility: Multiple interfaces offer flexibility since a class can implement any number of interfaces, each with distinct functionality.
Disadvantages of Multiple Hierarchical Inheritance
Complexity: With the use of multiple interfaces, the structure of the code may become more complex, especially if the interfaces have overlapping methods.
No State Inheritance: Unlike classes, interfaces cannot have instance fields. Therefore, a class implementing multiple interfaces does not inherit any state (i.e., variables) from the interfaces.
Key Points to Remember
To prevent confusion, Java does not allow multiple inheritance with classes.
Multiple Hierarchical Inheritance can be achieved using interfaces, where a class implements multiple interfaces.
Interfaces allow defining a contract for behavior but do not include state (fields).
Java allows you to simulate multiple inheritance by implementing multiple interfaces in a class.
Conclusion
Java inheritance is a powerful concept, even though it might initially seem complex. By leveraging interfaces, Java allows classes to inherit behavior from multiple sources, enabling a flexible and modular approach to designing software. Understanding how to use interfaces effectively will help you manage multiple hierarchies in your Java applications while avoiding the pitfalls of ambiguity that could arise in other languages with direct multiple inheritance.
Comments