Understanding Hierarchical Inheritance in Java: Concepts and Examples
- shakyapreeti650
- Dec 31, 2024
- 4 min read
Introduction
Inheritance is one of the core concepts in object-oriented programming (OOP), allowing a class to acquire properties and behaviors (methods) from another class. In Java, inheritance helps in reusing the code and establishing a relationship between parent and child classes. One specific type of inheritance is Hierarchical Inheritance, where multiple child classes inherit from a single-parent class. This article will discuss hierarchical inheritance in Java with an example program to help you understand its implementation and significance.
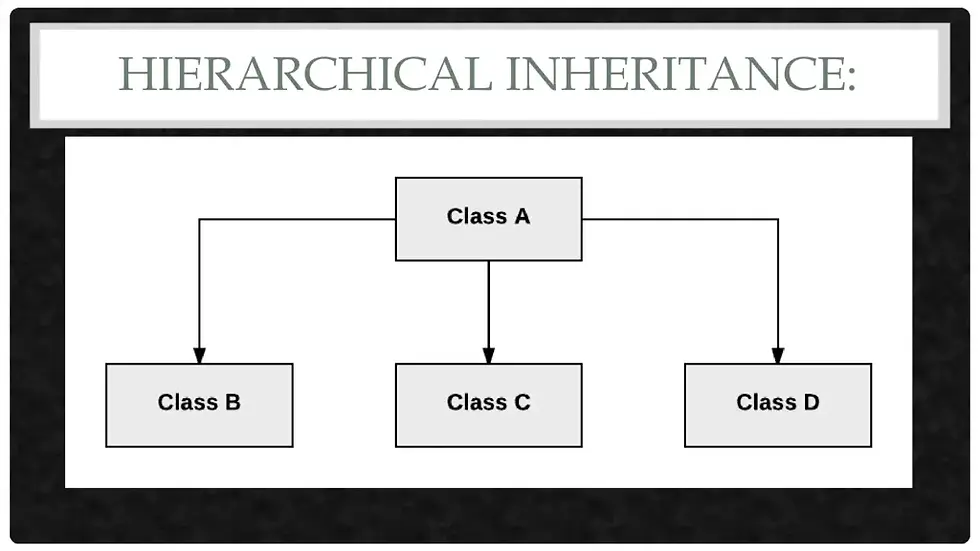
What is Hierarchical Inheritance in Java?
In Hierarchical Inheritance, one parent class is inherited by multiple child classes. The child classes can reuse the code from the parent class without needing to rewrite the same methods and properties. Each child class can also have its unique attributes and behaviors, in addition to inheriting those from the parent class.
For example:
A Vehicle class (parent class) can be inherited by Car, Bike, and Truck (child classes), where all child classes will inherit common properties like speed, fuel, and methods like start() and stop(), while also having their own unique features.
Advantages of Hierarchical Inheritance:
Code Reusability: The child classes can reuse the properties and methods of the parent class, leading to reduced code duplication.
Extensibility: New child classes can be added easily to the existing hierarchy, making the system extensible.
Maintainability: Since common code resides in the parent class, updates to that code can be made in one place without affecting the child classes.
Hierarchical Inheritance Syntax in Java
The syntax for implementing hierarchical inheritance in Java is straightforward. Here’s the basic structure:
java
Copy code
class ParentClass {
// Parent class properties and methods
}
class ChildClass1 extends ParentClass {
// Additional properties and methods specific to ChildClass1
}
class ChildClass2 extends ParentClass {
// Additional properties and methods specific to ChildClass2
}
The ParentClass is inherited by multiple child classes like ChildClass1 and ChildClass2, all of which inherit its properties and methods.
Example of Hierarchical Inheritance in Java
Let’s consider an example where we have a parent class Animal with common properties like name and, sound, and two child classes Dog and Cat that inherit from the Animal.
java
Copy code
// Parent class
class Animal {
String name;
String sound;
// Constructor
public Animal(String name, String sound) {
this.name = name;
this.sound = sound;
}
// Method to display animal details
public void displaying() {
System.out.println("Name: " + name);
System.out.println("Sound: " + sound);
}
}
// Child class 1
class Dog extends Animal {
// Constructor of Dog class
public Dog(String name, String sound) {
super(name, sound); // Calling parent class constructor
}
// Additional method specific to Dog
public void fetch() {
System.out.println(name + " loves to fetch the ball!");
}
}
// Child class 2
class Cat extends Animal {
// Constructor of Cat class
public Cat(String name, String sound) {
super(name, sound); // Calling parent class constructor
}
// Additional method specific to Cat
public void scratch() {
System.out.println(name + " loves to scratch furniture!");
}
}
public class Main {
public static void main(String[] args) {
// Creating objects of Dog and Cat
Dog dog = new Dog("Buddy", "Bark");
Cat cat = new Cat("Whiskers", "Meow");
// Calling methods from the parent class and child classes
System.out.println("Dog Info:");
dog.displayInfo();
dog.fetch();
System.out.println("\nCat Info:");
cat.displayInfo();
cat.scratch();
}
}
Explanation of the Program:
Animal Class (Parent Class):
It has two properties: name and sound, which are common to all animals.
The constructor initializes these properties, and the displayInfo() method prints the animal’s details.
Dog Class (Child Class):
It inherits the Animal class using the extends keyword.
The constructor of Dog class calls the parent class constructor using super(name, sound) to initialize the name and sound properties.
The fetch() method is specific to the Dog class, allowing dogs to perform an action unique to them.
Cat Class (Child Class):
It inherits the Animal class in the same way as Dog.
The scratch() method is specific to the Cat class.
Main Class:
This class creates instances of the Dog and Cat classes and demonstrates the inheritance by calling the displayInfo() method (inherited from Animal) and their respective specific methods (fetch() for Dog and scratch() for Cat).
Output:
vbnet
Copy code
Dog Info:
Name: Buddy
Sound: Bark
Buddy loves to fetch the ball!
Cat Info:
Name: Whiskers
Sound: Meow
Whiskers loves to scratch the furniture!
Key Points:
Code Reusability: Both Dog and Cat reuse the code from the Animal class (i.e., the name and sound properties and the displayInfo() method).
Method Overriding: If the child class wants to provide a different implementation of a method (for example, a method speak() in the parent class), the method can be overridden in the child class.
When to Use Hierarchical Inheritance?
Hierarchical inheritance is useful in scenarios where you have multiple classes sharing common functionality but also require distinct behavior. Here are a few examples:
Vehicle System: Different types of vehicles (car, truck, motorcycle) can inherit from a Vehicle class and share common properties like speed and fuel, while having their own unique behavior (e.g., Car can have a roof type property, Truck can have a cargo capacity property).
Animal Kingdom: All animals can inherit from a common Animal class, sharing properties like name and sound, while having unique behaviors, such as fly() for birds and swim() for fish.
Conclusion
Hierarchical Inheritance in Java allows a parent class to be inherited by multiple child classes, promoting code reusability and extensibility. The child classes inherit common attributes and methods from the parent, while also defining their own specific behavior. By understanding Hierarchical Inheritance in Java Example, you can design systems where different entities share common functionality while maintaining their own unique properties and methods.
This type of inheritance is beneficial in scenarios where different classes share common behavior but also need to implement unique actions or features. For instance, a parent class can define common properties, and child classes can extend those properties with specific characteristics or actions.
Comments